Add User Data
Integrate our JavaScript SDK to easily link user feedback on Supahub to your existing user data.
Add user data
Integrate our JavaScript SDK to effortlessly synchronize feedback submitted on Supahub with users’ existing accounts in your app.
By including user data such as name, email, avatar, etc., you can gather more detailed context about your users.
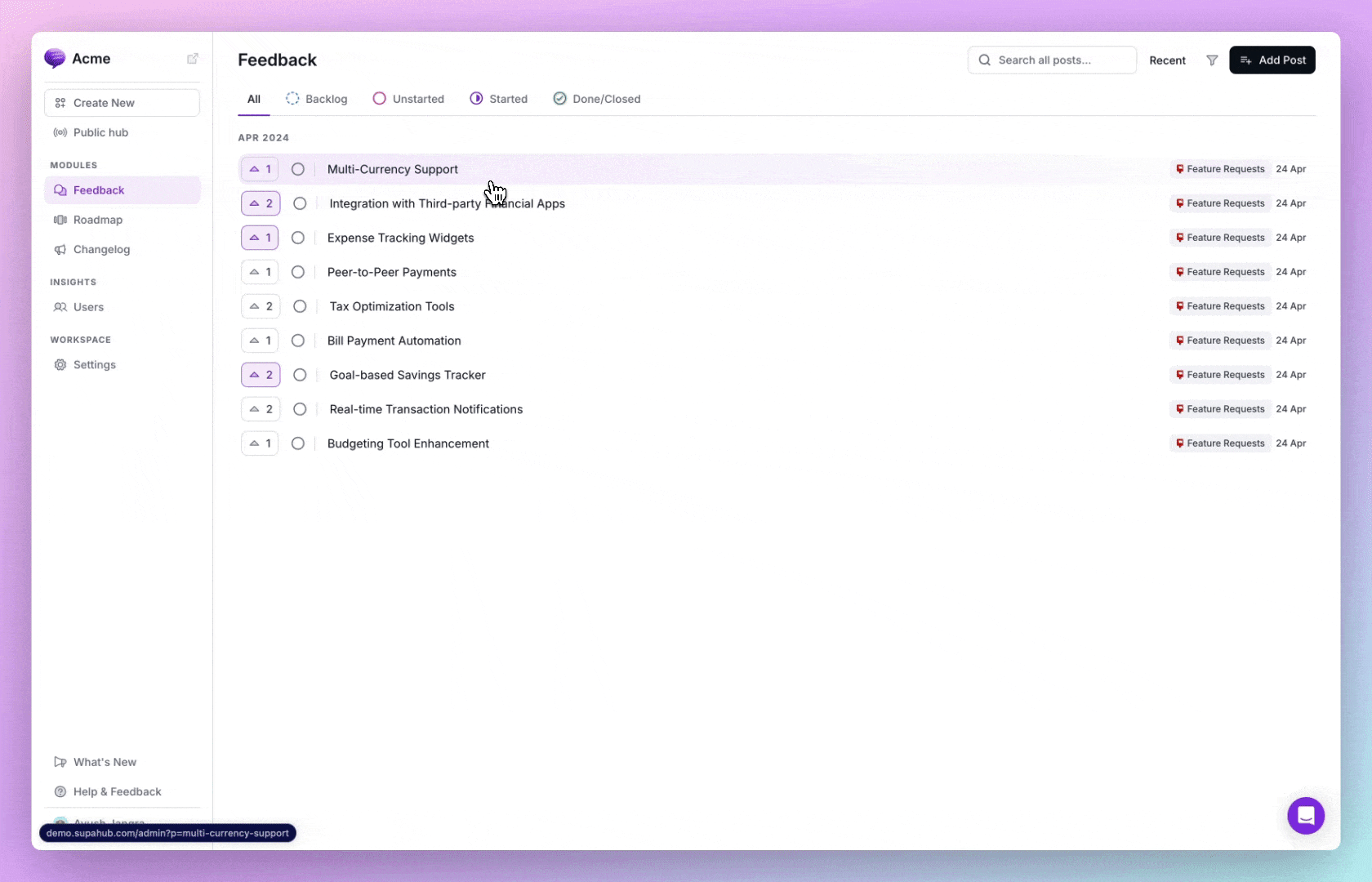
To begin using our SDK, insert the following code snippet into your app:
Please keep in mind that the Supahub Identify feature allows you to send user data from your app to Supahub for a better understanding of user behavior. If you want a feature that allows your users to log in seamlessly to Supahub without having to log in separately, consider implementing Single Sign-On (SSO).
Add custom fields for a user
You can enhance the information associated with your users and companies by adding custom fields, such as their title, location, and more. By utilizing this feature, you can further segment and analyze your user feedback data in Supahub.
To add custom data to an identify call, follow this example:
Although optional, we strongly suggest including the user’s company information as it allows you to sort posts based on user’s monthly spend and other company data. Add Company Data
Please note that the Supahub Identify feature does not identify admins. When testing, it is recommended to use a non-admin account.